Basic usage
This tutorial will guide you through effectively using FileBrowserView in your personal projects. Not every feature will be covered here, but the source code is packed with Javadocs/comments to help you get the most out of the library.
Adding the library to your project
You can download the library as a JAR file (recommended) or fork the entire project source into Android Studio. Once you've downloaded the library, add it to your project as a module.
Adding the view to your layouts
You can add FileBrowserView to any layout within an Activity or a Fragment. FileBrowserView is an extension of Android's built-in FrameLayout. You can add it as a child view within a ViewGroup or as a parent ViewGroup within an Activity or Fragment.
<!-- View declaration. You can use all standard view attributes here. -->
<com.psaravan.filebrowserview.lib.View.FileBrowserView
android:id="@+id/fileBrowserView"
android:layout_height="wrap_content"
android:layout_width="match_parent" />
Getting a basic version of FileBrowserView up and running
First, create an instance of FileBrowserView within the Activity or Fragment where you added the view:
FileBrowserView fileBrowserView = (FileBrowserView) findViewById(R.id.fileBrowserView);
You can now customize your view using the FileBrowserView instance you just created. Here's an example that shows how to set up and initialize a basic version of the view:
//Customize the view.
fileBrowserView.setFileBrowserLayoutType(FileBrowserView.FILE_BROWSER_LIST_LAYOUT) //Set the type of view to use.
.setDefaultDirectory(new File("/")) //Set the default directory to show.
.setShowHiddenFiles(true) //Set whether or not you want to show hidden files.
.showItemSizes(true) //Shows the sizes of each item in the list.
.showOverflowMenus(true) //Shows the overflow menus for each item in the list.
.init(); //Loads the view. You MUST call this method, or the view will not be displayed.
And here's the result:
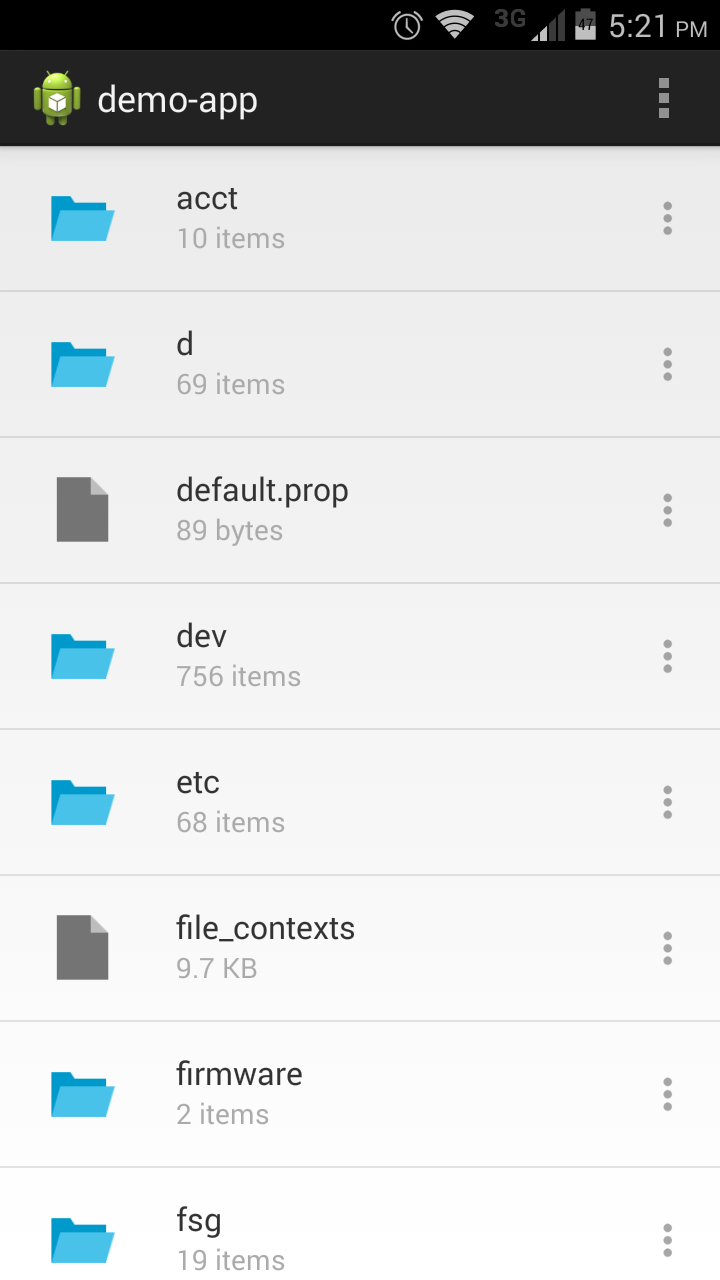
Here are a few important points to note:
- The
init()
method is what actually displays the view to the user. You MUST call this method or the view will not be visible. - If you set/change any options for the view after calling the
init()
method, you must callinit()
again to display the changes.
Customization
This section will cover some of the customization options and that FileBrowserView has to offer. You can mix and match most of these options to tailor the library to your needs.
Customizing the default adapters
The library comes with two different adapters by default (one each for the ListView and GridView representation). You can customize these default adapters to a limited extent, using the following built-in methods (for complete control on customizing the adapters, check out the next section):
showOverflowMenus()
: Allows you to show or hide the overflow menu icons in the default adapters. The overflow menus allow the user to access functionality such as copy, cut, paste, and delete. If you don't call this method and pass false, the overflow menus will be shown by default.showItemSizes()
: Allows you to show or hide the size of each item under its name. For files, the size is displayed as a formatted string (3 MB, 44 KB, 6 GB, etc.). For folders, the size is displayed as the number of items within that folder (3 items, 56 items, etc.).showItemIcons()
: Allows you to show or hide the icon next to each item name.
Using your own adapter
If you want to use a completely different layout for each list/grid item or want to customize how the view elements look, you'll have to create your own adapter.
Your custom adapter MUST extend AbstractFileBrowserAdapter.
If you do not use a subclass of AbstractFileBrowserAdapter, your app will crash with an IllegalArgumentException. Once you create an adapter class that extends AbstractFileBrowserView,
make sure you override the getView()
method. Just like a regular data adapter, this is where you can customize the view and logic for each item in the list/grid.
You should also override the onOverflowClick()
method. If your list/grid will display an overflow menu button for each item, call this method when the button is clicked.
If your list/grid won't have an overflow menu button, simply override the method and leave its body empty.
AbstractFileBrowserAdapter comes with a set of methods that expose the details of each file/folder item. Each method returns an ArrayList with individual details on all the items in the list/grid. These ArrayLists are parallel lists, so you can grab all the details of an individual item by fetching the data at the same index in each list:
getNamesList()
: Returns an ArrayList of file/folder names within the current directory.getSizesList()
: Returns an ArrayList of file/folder sizes within the current directory. File sizes are stored as formatted strings (3 MB, 44 KB, 6 GB, etc.) while folder sizes are stored as the number of items within that folder (3 items, 56 items, etc.).getTypesList()
: Returns an ArrayList of file/folder types within the current directory. The file/folder types are stored as Integer flags from the FileBrowserEngine class (FILE_AUDIO
,FILE_PICTURE
,FILE_VIDEO
,FILE_GENERIC
,FOLDER
).getPathsList()
: Returns an ArrayList of file/folder paths within the current directory. The paths are stored as canonical path strings.
You can now use these lists to display data about each individual file/folder in your list/grid item. For example,
here's a code snippet that shows you how to implement the getView()
method with the lists:
@Override
public View getView(int position, View convertView, ViewGroup parent) {
FoldersViewHolder holder = null;
if (convertView == null) {
convertView = LayoutInflater.from(mContext).inflate(R.layout.list_view_item, parent, false);
FoldersViewHolder holder = new FoldersViewHolder();
holder.title = (TextView) convertView.findViewById(R.id.title);
}
//Grab the name of the item.
String name = getNamesList().get(position);
//Display the name to the user using the TextView.
holder.title.setText(name);
}
Check out the default ListView adapter and the default GridView adapter for a complete example on how to build one. You can also go ahead and just use these adapters if they have everything you need.
Filesystem operations
FileBrowserView supports basic filesystem operations such as copying, deleting, moving, and renaming files and folders. Batch operations are currently not supported but will be added in the near future. All operations are performed with AsyncTasks and will run on their own indvidual threads.
If you are using the default adapters, the item overflow menus already have options for Copy, Move, Rename, and Delete. However, you'll have to manually implement your own logic for each filesystem operaiton by calling the following AsyncTasks.
Copying
You'll need two standard File
objects: one for the source file and another one for the destination file.
You can also specify if you want to display an indeterminate progress dialog while the operation takes place.
Here's how to execute the copy operation:
//Create the source file and destination file.
File sourceFile = new File("/sdcard/test.db");
File destFile = new File("/sdcard/CopyFolder/test.db");
//This command will copy /sdcard/test.db to /sdcard/CopyFolder/test.db while displaying a progress dialog.
AsyncCopyTask task = new AsyncCopyTask(context, sourceFile, destFile, true);
task.execute();
A toast message will be shown once the operation completes.
Moving/Renaming
Just like the copy operation, you'll need two standard File
objects: one for the source file and another one for the destination file.
You can also specify if you want to display an indeterminate progress dialog while the operation takes place.
Here's how to execute the move operation:
//Create the source file and destination file.
File sourceFile = new File("/sdcard/test.db");
File destFile = new File("/sdcard/CopyFolder/test.db");
//This command will move /sdcard/test.db to /sdcard/CopyFolder/test.db while displaying a progress dialog.
AsyncMoveTask task = new AsyncMoveTask(context, sourceFile, destFile, true);
task.execute();
A toast message will be shown once the operation completes.
Deleting
You'll only need one standard File
object for this operation: the file you want to delete.
You can also specify if you want to display an indeterminate progress dialog while the operation takes place.
Here's how to execute the delete operation:
//Create the source file.
File sourceFile = new File("/sdcard/test.db");
//This command will delete /sdcard/test.db while displaying a progress dialog.
AsyncDeleteTask task = new AsyncDeleteTask(context, sourceFile, true);
task.execute();
A toast message will be shown once the operation completes.
Interfaces
FileBrowserView provides a multitude of interfaces that allow you to tightly integrate the view into your app. Each interface and callback method is thoroughly documented with Javadocs, so you can check out the Interfaces package to learn how to use each one.